API Access
A web API endpoint for executing DSL queries is available. The Python script below illustrates how to make a DSL query using the web API.
Note
The Dimensions Analytics API is limited to 30 requests per IP address per minute.
Python : raw access
The following code snippet shows how to connect to the Dimensions API only using Python’s requests library.
import requests
# The credentials to be used
login = {
'key': "your API key"
}
# Send credentials to login url to retrieve token. Raise
# an error, if the return code indicates a problem.
# Please use the URL of the system you'd like to access the API
# in the example below.
resp = requests.post('https://<your-url.dimensions.ai>/api/auth.json', json=login)
resp.raise_for_status()
# Create http header using the generated token.
headers = {
'Authorization': "JWT " + resp.json()['token']
}
# Execute DSL query.
resp = requests.post(
'https://<your-url.dimensions.ai>/api/dsl.json',
data='search publications return publications'.encode(),
headers=headers)
# Display raw result
print(resp.json())
The snippet above uses the key authentication method, which is the primary method for most API servers (see also this page for more information on how to obtain a key).
Some legacy users may still have to authenticate using their username and password. If that’s the case, simply replace the beginning of the snippet above as follows:
login = {
'username': 'your username',
'password': 'your password'
}
Note
Please note escaping rules in Python. For example, when writing a query with escaped quotes, such as:
search publications for "\"phrase 1\" AND \"phrase 2\""
in Python, it is necessary to escape the backslashes as well, so it would look like:
resp = requests.post(
'https://<your-url.dimensions.ai>/api/dsl.json',
data='search publications for "\\"phrase 1\\" AND \\"phrase 2\\""',
headers=headers)
Similar escaping rules might apply to other programming languages than Python as well.
Python : Dimcli
Dimensions APIs can be accessed also using Dimcli, an open-source Python client. Dimcli is a convenient way to connect to, query and analyze the results of Dimensions API.
Dimcli includes also a command line interface (CLI) that aims at simplifying the process of learning the grammar of the Dimensions Search Language. Calling dimcli
from a Terminal opens an interactive query console with syntax autocompletion, persistent history across sessions, pretty-printing and preview of JSON results, export to HTML and CSV, and more.
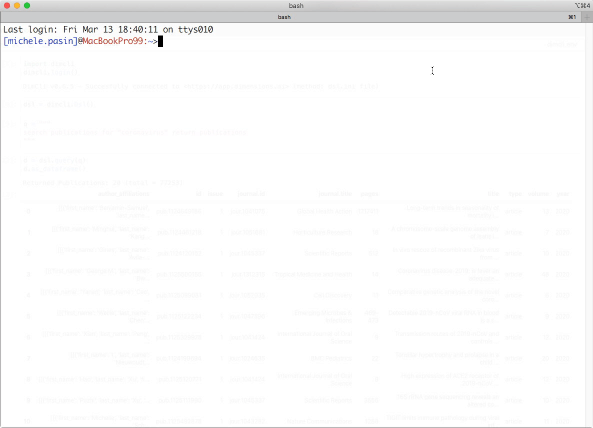
For further details regarding this package, please consult the Getting started with Dimcli page.
curl + jq
This example shows a minimal way to query the API. This should be easily transferable to any other programming language or environment.
export DSL_TOKEN=$(curl https://<your-url.dimensions.ai>/api/auth.json -d '{"username": "your username", "password": "your password"}' -s|jq -r .token)
curl https://<your-url.dimensions.ai>/api/dsl.json -H "Authorization: JWT $DSL_TOKEN" -d 'search publications return publications' -s|jq
Note
Note that jq tool is used to extract a “token” value from the JSON response in the first request. In the second request, jq is used only to pretty print the result so it’s use is optional.
PowerShell
This is an equivalent example of the above CURL + JQ Example, but usable in the PowerShell enivornment.
$DSL_TOKEN = (Invoke-RestMethod -Uri https://<your-url.dimensions.ai>/api/auth.json -Method Post -Body (@{'username' = 'your username'; 'password' = 'your password'} | ConvertTo-JSON)).token
Invoke-RestMethod -Uri https://<your-url.dimensions.ai>/api/dsl.json -Method Post -H @{Authorization = "JWT $DSL_TOKEN"} -Body 'search publications return publications'
Postman
This examples shows how to set up Postman to query the Dimensions API. This can be achieved in two simple steps.
1. Obtaining a JWT token
Make a POST request to https://app.dimensions.ai/api/auth.json
with body { "username": "<your-username>", "password": "<your-password>"}
This will return a JWT token, which should be noted as it will be used in the next step.
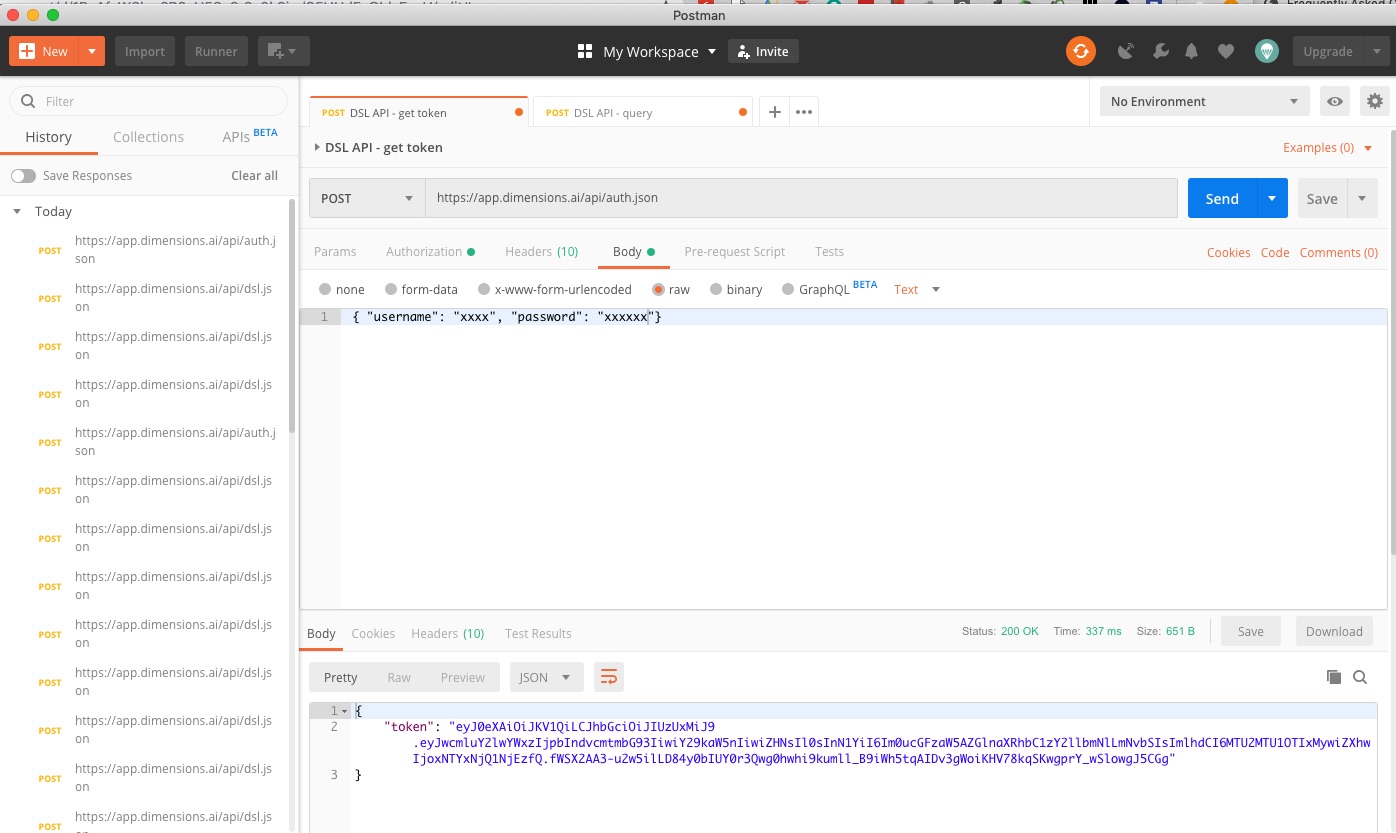
2. Using the token to query the API
Make a new POST request by adding the token to the Headers panel in this format: Authorization : JWT {theToken}.
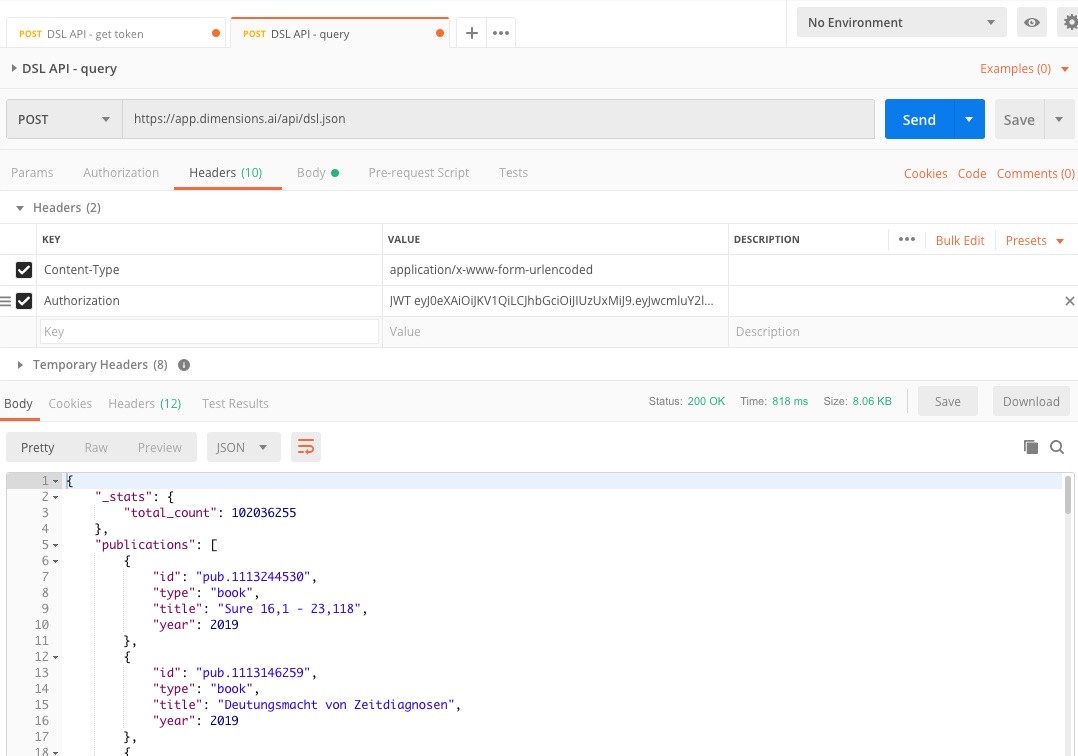
Finally, Compose your query in the request body section:
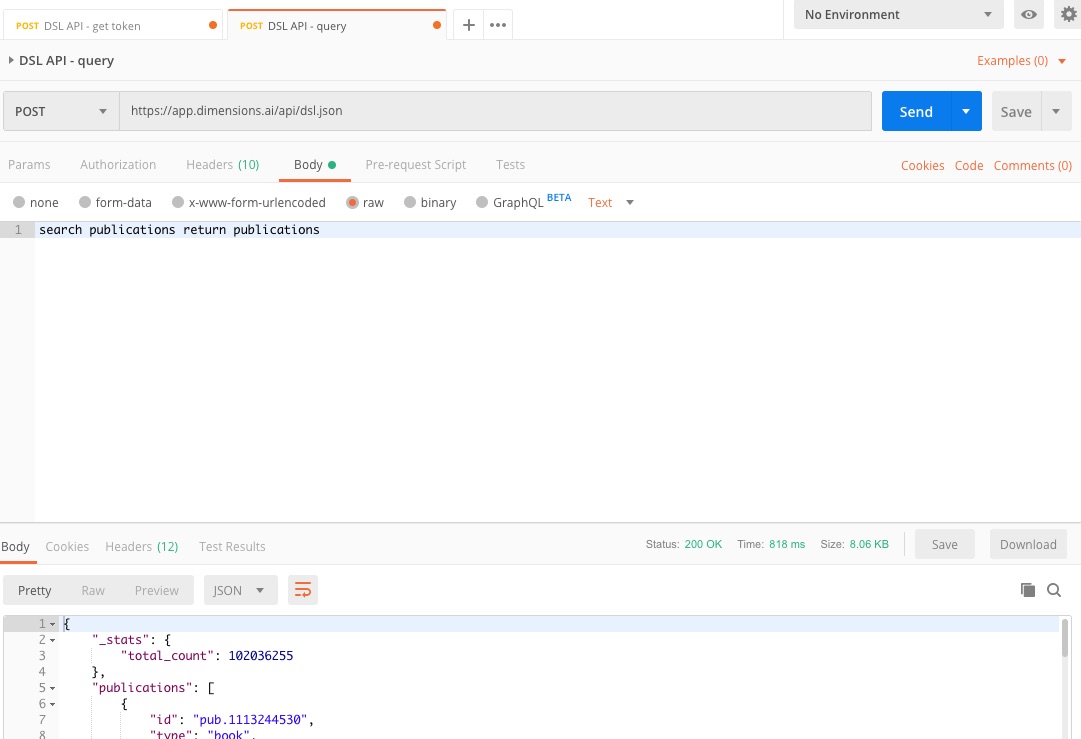
Note
Another approach to get started with Postman is by using the cURL importer.
Third-party libraries
- dimensionsR Library
The dimensionsR library is an open-source R-package to gather bibliographic data using the Dimensions API and the DSL.
- rdimensions Library
Another client library rdimensions for interacting with the Dimensions API from the R environment.
- Sidewall Python Library
The Sidewall library is a Python package providing object classes for Dimensions entities, fetches data incrementally, caches results, copes with rate limits, and more, to make working with Dimensions in Python more natural.